Error Handling
Part 02 - Error Handling
Error Handling and Status Page Error Handling
It's important to handle errors in a meaningful and user-friendly way, as well as to log errors for debugging and troubleshooting purposes. Proper error handling can greatly improve the stability and reliability of your ASP.NET application.
ExceptionController
using BPX.Website.Models;
using Microsoft.AspNetCore.Diagnostics;
using Microsoft.AspNetCore.Mvc;
namespace BPX.Website.Controllers
{
public class ExceptionController : BaseController<ExceptionController>
{
public ExceptionController(ILogger<ExceptionController> logger) : base(logger)
{
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Index(int? statusCode = null)
{
string errorStatusCode = string.Empty;
string errorMessage = string.Empty;
string errorURL = string.Empty;
if (statusCode != null)
{
var statusCodeReExecuteFeature = HttpContext.Features.Get<IStatusCodeReExecuteFeature>();
if (statusCodeReExecuteFeature is not null)
{
errorURL = string.Join(statusCodeReExecuteFeature.OriginalPathBase,
statusCodeReExecuteFeature.OriginalPath,
statusCodeReExecuteFeature.OriginalQueryString);
}
switch (statusCode.Value)
{
// 400 Bad Request
case 400:
errorStatusCode = statusCode.Value.ToString() + " Bad Request";
errorMessage = string.Join("Your request is not properly formed: ", errorURL);
break;
// 401 Unauthorized
case 401:
errorStatusCode = statusCode.Value.ToString() + " Unauthorized";
errorMessage = string.Join("The page you requested is no authorized: ", errorURL);
break;
// 403 Forbidden
case 403:
errorStatusCode = statusCode.Value.ToString() + " Forbidden";
errorMessage = string.Join("The page you requested is forbidden: ", errorURL);
break;
// 404 Not Found
case 404:
errorStatusCode = statusCode.Value.ToString() + " Not Found";
errorMessage = string.Join("The page you requested is not found: ", errorURL);
break;
// 405 Method Not Allowed
case 405:
errorStatusCode = statusCode.Value.ToString() + " Method Not Allowed";
errorMessage = string.Join("The method you requested is not allowed: ", errorURL);
break;
}
// code to log the exception
}
return View(new ErrorVM { ErrorStatusCode = errorStatusCode, ErrorMessage = errorMessage });
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
string errorMessage = string.Empty;
var exceptionFeature = HttpContext.Features.Get<IExceptionHandlerPathFeature>();
if (exceptionFeature != null)
{
// exception data from IExceptionHandlerPathFeature
// exceptionFeature.Path
// exceptionFeature.Error.Message
// exceptionFeature.Error.StackTrace
errorMessage = exceptionFeature.Error.Message;
// code to log the exception
}
return View(new ErrorVM { ErrorMessage = errorMessage });
}
}
}
Notes:none.
ErrorVM
namespace BPX.Website.Models
{
public class ErrorVM
{
public string? ErrorStatusCode { get; set; }
public string? ErrorMessage { get; set; }
}
}
Notes:none.
Exception - Index.cshtml
@model ErrorVM
@{
ViewData["Title"] = "Error Status Code";
}
<h1 class="text-danger">@Model.ErrorStatusCode</h1>
<h2 class="text-danger">An error occurred while processing your request.</h2>
<div>
@Model.ErrorMessage
</div>
Notes:none.
Shared - Error.cshtml
@model ErrorVM
@{
ViewData["Title"] = "Error";
}
<h1 class="text-danger">Error.</h1>
<h2 class="text-danger">An error occurred while processing your request.</h2>
<div>
@Model.ErrorMessage
</div>
Notes:none.
Program.cs
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllersWithViews();
var app = builder.Build();
// Configure the HTTP request pipeline.
app.UseExceptionHandler("/Exception/Error");
app.UseStatusCodePagesWithReExecute("/Exception/Index", "?statusCode={0}");
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
Notes:none
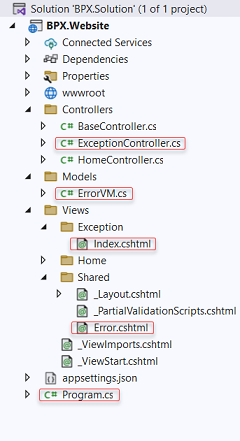
Ginger CMS
the future of cms, a simple and intuitive content management system ... ASP.NET MVC Application
best practices like Repository, LINQ, Dapper, Domain objects ... CFTurbine
cf prototyping engine, generates boilerplate code and views ... Search Engine LITE
create your own custom search engine for your web site ... JRun monitor
monitors the memory footprint of JRun engine and auto-restarts a hung engine ... Validation Library
complete validation library for your web forms ...