Domain & DbModels
Part 05 - Domain & DbModels
Domain & DbModels
BPX.Domain
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>net6.0</TargetFramework>
<ImplicitUsings>enable</ImplicitUsings>
<Nullable>enable</Nullable>
</PropertyGroup>
</Project>
Notes:none
Eatery.cs
using System.ComponentModel.DataAnnotations;
namespace BPX.Domain.DbModels
{
public class Eatery
{
public Eatery()
{
this.Name = string.Empty;
this.Address = string.Empty;
this.Phone = string.Empty;
this.StatusFlag = string.Empty;
}
[Key]
public int EateryId { get; set; }
[Required]
[StringLength(32)]
public string Name { get; set; }
[Required]
[StringLength(128)]
public string Address { get; set; }
[Required]
[StringLength(20)]
public string Phone { get; set; }
[Required]
[StringLength(1)]
public string StatusFlag { get; set; }
[Required]
public int ModifiedBy { get; set; }
[Required]
public DateTime ModifiedDate { get; set; }
}
}
Notes:none
Role.cs
using System.ComponentModel.DataAnnotations;
namespace BPX.Domain.DbModels
{
public class Role
{
public Role()
{
this.RoleName = string.Empty;
this.StatusFlag = string.Empty;
}
[Key]
public int RoleId { get; set; }
[Required]
[StringLength(32)]
public string RoleName { get; set; }
[Required]
[StringLength(1)]
public string StatusFlag { get; set; }
[Required]
public int ModifiedBy { get; set; }
[Required]
public DateTime ModifiedDate { get; set; }
}
}
Notes:none
User.cs
using System.ComponentModel.DataAnnotations;
namespace BPX.Domain.DbModels
{
public class User
{
public User()
{
this.FirstName = string.Empty;
this.LastName = string.Empty;
this.Email = string.Empty;
this.Mobile = string.Empty;
this.UserLogin = string.Empty;
this.UserPassword = string.Empty;
this.StatusFlag = string.Empty;
}
[Key]
public int UserId { get; set; }
[Required]
[StringLength(32)]
public string FirstName { get; set; }
[Required]
[StringLength(32)]
public string LastName { get; set; }
[Required]
[EmailAddress]
[StringLength(64)]
public string Email { get; set; }
[StringLength(20)]
public string Mobile { get; set; }
[Required]
[StringLength(16)]
public string UserLogin { get; set; }
[Required]
[StringLength(32)]
public string UserPassword { get; set; }
[Required]
[StringLength(1)]
public string StatusFlag { get; set; }
[Required]
public int ModifiedBy { get; set; }
[Required]
public DateTime ModifiedDate { get; set; }
}
}
Notes:none
UserRole.cs
using System.ComponentModel.DataAnnotations;
namespace BPX.Domain.DbModels
{
public class UserRole
{
public UserRole()
{
this.StatusFlag = string.Empty;
}
//Composite keys can only be configured using the Fluent API; conventions will never
//set up a composite key, and you can not use Data Annotations to configure one.
//[Key]
[Required]
public int UserId { get; set; }
//[Key]
[Required]
public int RoleId { get; set; }
[Required]
[StringLength(1)]
public string StatusFlag { get; set; }
[Required]
public int ModifiedBy { get; set; }
[Required]
public DateTime ModifiedDate { get; set; }
}
}
Notes:none
DDL Script for reference
CREATE TABLE Users (
UserId int IDENTITY(1,1) NOT NULL,
FirstName varchar(32) NOT NULL,
LastName varchar(32) NOT NULL,
Email varchar(64) NOT NULL,
Mobile varchar(20) NULL,
UserLogin varchar(16) NOT NULL,
UserPassword varchar(32) NOT NULL,
StatusFlag char(1) NOT NULL,
ModifiedBy int NOT NULL,
ModifiedDate datetime NOT NULL,
PRIMARY KEY CLUSTERED (UserId ASC),
CONSTRAINT UC_Users_Email UNIQUE (Email)
);
CREATE TABLE Roles (
RoleId int IDENTITY(1,1) NOT NULL,
Name varchar(32) NOT NULL,
StatusFlag char(1) NOT NULL,
ModifiedBy int NOT NULL,
ModifiedDate datetime NOT NULL,
PRIMARY KEY CLUSTERED (RoleId ASC),
CONSTRAINT UC_Roles_Name UNIQUE (Name)
);
CREATE TABLE UserRoles (
UserId int NOT NULL,
RoleId int NOT NULL,
StatusFlag char(1) NOT NULL,
ModifiedBy int NOT NULL,
ModifiedDate datetime NOT NULL,
PRIMARY KEY CLUSTERED (UserId, RoleId),
CONSTRAINT FK_UserRoles_UserId FOREIGN KEY (UserId) REFERENCES Users (UserId),
CONSTRAINT FK_UserRoles_RoleId FOREIGN KEY (RoleId) REFERENCES Roles (RoleId)
);
CREATE TABLE Eaterys (
EateryId int IDENTITY(1,1) NOT NULL,
Name varchar(32) NOT NULL,
Address varchar(128) NOT NULL,
Phone varchar(20) NULL,
StatusFlag char(1) NOT NULL,
ModifiedBy int NOT NULL,
ModifiedDate datetime NOT NULL,
PRIMARY KEY CLUSTERED (EateryId ASC)
);
Notes:none
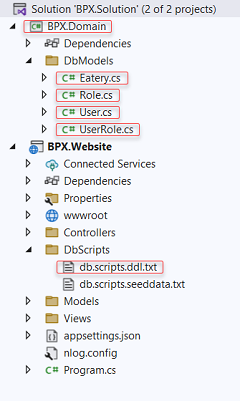
Ginger CMS
the future of cms, a simple and intuitive content management system ... ASP.NET MVC Application
best practices like Repository, LINQ, Dapper, Domain objects ... CFTurbine
cf prototyping engine, generates boilerplate code and views ... Search Engine LITE
create your own custom search engine for your web site ... JRun monitor
monitors the memory footprint of JRun engine and auto-restarts a hung engine ... Validation Library
complete validation library for your web forms ...