DAL & Test DAL
Part 06 - DAL & Test DAL
DAL & Test DAL
xxx
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>net6.0</TargetFramework>
<ImplicitUsings>enable</ImplicitUsings>
<Nullable>enable</Nullable>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Microsoft.EntityFrameworkCore" Version="7.0.2" />
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer" Version="7.0.2" />
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\BPX.Domain\BPX.Domain.csproj" />
</ItemGroup>
</Project>
Notes:none
xxx
install Microsoft.EntityFrameworkCore (latest version) using NuGet Package Manager
Notes:none
xxx
install Microsoft.EntityFrameworkCore.SqlServer (latest version) using NuGet Package Manager
Notes:none
xxx
add project reference to BPX.Domain library
Notes:none
xxx
using BPX.Domain.DbModels;
using Microsoft.EntityFrameworkCore;
namespace BPX.DAL.Context
{
public partial class EFContext : DbContext
{
public EFContext(DbContextOptions<EFContext> options) : base(options)
{
}
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<UserRole>().HasKey(m => new { m.UserId, m.RoleId });
}
public virtual DbSet<User> Users { get; set; }
public virtual DbSet<Role> Roles { get; set; }
public virtual DbSet<UserRole> UserRoles { get; set; }
public virtual DbSet<Eatery> Eaterys { get; set; }
}
}
Notes:none
xxx
add project reference to BPX.DAL libraries
Notes:none
xxx
add project reference to BPX.Domain libraries
Notes:none
xxx
using BPX.DAL.Context;
using Microsoft.AspNetCore.Mvc;
namespace BPX.Website.Controllers
{
public class TestController : BaseController<TestController>
{
private readonly EFContext efContext;
public TestController(ILogger<TestController> logger, EFContext efContext) : base(logger)
{
this.efContext = efContext;
}
public IActionResult Index()
{
var rslt1 = efContext.Users.ToList();
var rslt2 = efContext.Roles.ToList();
var rslt3 = efContext.UserRoles.ToList();
var rslt4 = efContext.Eaterys.ToList();
return View();
}
}
}
Notes:none
xxx
{
"Logging": {
"LogLevel": {
"Default": "Trace",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*",
"ConnectionStrings": {
"BPXConnectionString": "Server=...;Database=...;User Id=...;Password=...;Integrated Security=...;MultipleActiveResultSets=...;Encrypt=...;TrustServerCertificate=...;"
}
}
Notes:none
xxx
using BPX.DAL.Context;
using Microsoft.EntityFrameworkCore;
using NLog;
using NLog.Web;
var logger = NLog.LogManager.Setup().LoadConfigurationFromAppSettings().GetCurrentClassLogger();
logger.Debug("init main");
try
{
var builder = WebApplication.CreateBuilder(args);
var connectionString = builder.Configuration.GetConnectionString("BPXConnectionString");
builder.Services.AddDbContext<EFContext>(x => x.UseSqlServer(connectionString));
// Add services to the container.
builder.Services.AddControllersWithViews();
// NLog: Setup NLog for Dependency injection
builder.Logging.ClearProviders();
builder.Host.UseNLog();
var app = builder.Build();
// Configure the HTTP request pipeline.
app.UseExceptionHandler("/Exception/Error");
app.UseStatusCodePagesWithReExecute("/Exception/Index", "?statusCode={0}");
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
}
catch (Exception exception)
{
// NLog: catch setup errors
logger.Error(exception, "Stopped program because of exception");
throw;
}
finally
{
// Ensure to flush and stop internal timers/threads before application-exit (Avoid segmentation fault on Linux)
NLog.LogManager.Shutdown();
}
Notes:none
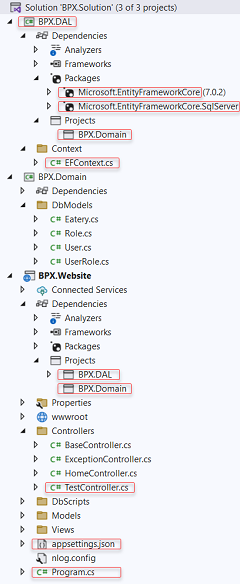
Ginger CMS
the future of cms, a simple and intuitive content management system ... ASP.NET MVC Application
best practices like Repository, LINQ, Dapper, Domain objects ... CFTurbine
cf prototyping engine, generates boilerplate code and views ... Search Engine LITE
create your own custom search engine for your web site ... JRun monitor
monitors the memory footprint of JRun engine and auto-restarts a hung engine ... Validation Library
complete validation library for your web forms ...